Remove Duplication from Sorted Linked List
Given the head
of a sorted linked list, delete all duplicates such that each element appears only once. Return the linked list sorted as well.
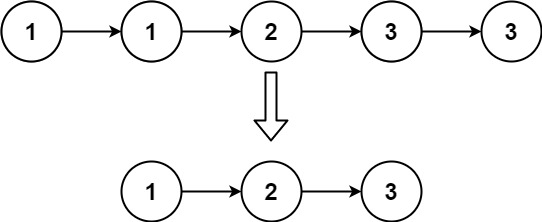
var deleteDuplicates = function(head) {
if (!head) {
return head;
}
let pre = head;
let cur = head.next;
while (cur) {
if (pre.val === cur.val) {
pre.next = cur.next;
cur = cur.next;
} else {
pre=pre.next;
cur=cur.next;
}
}
return head;
};